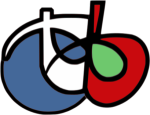 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbLabelObjectToPolygonFunctor_h
22 #define otbLabelObjectToPolygonFunctor_h
61 template <
class TLabelObject,
class TPolygon>
71 typedef typename LabelObjectType::LineType
LineType;
89 return "LabelObjectToPolygonFunctor";
249 #ifndef OTB_MANUAL_INSTANTIATION
std::vector< IndexType > IndexVectorType
Internal enums.
const SpacingType & GetSpacing() const
RunsPerLineVectorType m_InternalDataSet
Internal enums.
PolygonType::Pointer PolygonPointerType
IndexType LeftMostRightEndInside(unsigned int line, const IndexType &point, const IndexType &run) const
void SetOrigin(const PointType &origin)
PolygonType::VertexType VertexType
PositionFlagType m_PositionFlag
The position flag.
void WalkLeft(unsigned int line, const IndexType &startPoint, const IndexType &endPoint, PolygonType *polygon, const StateType state)
Walk left to update the finite states machine.
LabelObjectType::ConstLineIterator ConstLineIteratorType
bool IsRunIndexValid(const IndexType &index) const
Check if the given run index (index in line, line) is valid.
This class vectorizes a LabelObject to a Polygon.
RegionIndexType m_StartIndex
Internal enums.
IndexType m_CurrentPoint
The current point for vectorization.
VertexType IndexToPoint(const VertexType &index) const
Internal enums.
static bool LexicographicalLineCompare(const LineType &l1, const LineType &l2)
Compare two line in the lexicographical order.
void SetSpacing(const SpacingType &spacing)
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
int m_CurrentLine
The current line for vectorization.
virtual ~LabelObjectToPolygonFunctor()
void WalkRight(unsigned int line, const IndexType &startPoint, const IndexType &endPoint, PolygonType *polygon, const StateType state)
Walk right to update the finite states machine.
const PointType & GetOrigin() const
IndexType m_StartingPoint
Internal enums.
PositionFlagType
Internal enums.
std::vector< RunsPerLineType > RunsPerLineVectorType
Internal enums.
PolygonType * operator()(LabelObjectType *labelObject)
LabelObjectToPolygonFunctor()
LineType::IndexType IndexType
LabelObjectType::LineType LineType
IndexType RightMostLeftEndInside(unsigned int line, const IndexType &point, const IndexType &run) const
TLabelObject LabelObjectType
PointType m_Origin
Internal enums.
IndexType Within(const IndexType &point, unsigned int line) const
Check if the point lies within the range of the line.
SpacingType m_Spacing
Internal enums.
itk::Point< double, 2 > PointType
void SetStartIndex(const RegionIndexType &index)
StateType m_CurrentState
The current state.
const char * GetNameOfClass()
IndexType RightEnd(const IndexType &runIndex) const
Return the right-end of the run.
itk::Index< 2 > RegionIndexType
IndexType LeftEnd(const IndexType &runIndex) const
Return the left-end of the run.
const RegionIndexType & GetStartIndex() const
PolygonPointerType m_Polygon
Internal enums.
std::vector< LineType > RunsPerLineType
Internal structures.
itk::Vector< double, 2 > SpacingType
IndexVectorType m_Solution
The vector of vectorized boundaries.
IndexType m_CurrentRun
The current run for vectorization.
unsigned int m_LineOffset
The line offset from start of the region.