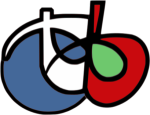 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbMaskedIteratorDecorator_h
22 #define otbMaskedIteratorDecorator_h
39 template <
typename TIteratorType,
typename TMaskIteratorType = TIteratorType>
44 typedef typename TMaskIteratorType::ImageType
MaskType;
45 typedef typename TIteratorType::ImageType
ImageType;
126 #ifndef OTB_MANUAL_INSTANTIATION
ImageType::RegionType RegionType
TMaskIteratorType & GetMaskIterator()
TIteratorType & GetImageIterator()
TMaskIteratorType::ImageType MaskType
void ComputeMaskedBegin()
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
ImageType::PixelType PixelType
MaskedIteratorDecorator(MaskType *mask, ImageType *image, const RegionType ®ion)
itkTypeMacroNoParent(MaskedIteratorDecorator)
Decorate an iterator to ignore masked pixels.
const bool & HasMask() const
MaskedIteratorDecorator< TIteratorType, TMaskIteratorType > Self
TIteratorType m_StartImage
MaskType::PixelType MaskPixelType
const PixelType & Value(void) const
ImageType::IndexType IndexType
TMaskIteratorType m_ItMask
TMaskIteratorType m_StartMask
IndexType GetIndex() const
TIteratorType::ImageType ImageType