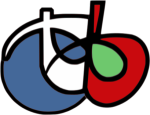 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbMeanShiftSegmentationFilter_h
22 #define otbMeanShiftSegmentationFilter_h
29 #include "itkRelabelComponentImageFilter.h"
30 #include "itkConnectedComponentFunctorImageFilter.h"
41 template <
class TInput>
50 return "ConnectedLabelFunctor";
56 return static_cast<bool>(p1 == p2);
70 void operator=(
const Self&) =
delete;
87 template <
class TInputImage,
class TOutputLabelImage,
class TOutputClusteredImage = TInputImage,
class TKernel = Meanshift::KernelUniform>
93 typedef itk::ImageToImageFilter<TInputImage, TOutputLabelImage>
Superclass;
112 itkStaticConstMacro(ImageDimension,
unsigned int, TInputImage::ImageDimension);
185 void GenerateData()
override;
197 #ifndef OTB_MANUAL_INSTANTIATION
RegionMergingFilterType::Pointer RegionMergingFilterPointerType
itk::SmartPointer< Self > Pointer
itk::SmartPointer< Self > Pointer
OutputClusteredImageType MeanShiftFilteredImageType
RelabelComponentFilterPointerType m_RelabelFilter
RegionPruningFilterPointerType m_RegionPruningFilter
MeanShiftSegmentationFilter Self
itk::SmartPointer< const Self > ConstPointer
LabelImageRegionPruningFilter< OutputLabelImageType, OutputClusteredImageType, OutputLabelImageType, OutputClusteredImageType > RegionPruningFilterType
itk::ConnectedComponentFunctorImageFilter< InputLabelImageType, InputLabelImageType, LabelFunctorType > RelabelComponentFilterType
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
Creation of an "otb" image which contains metadata.
RegionMergingFilterPointerType m_RegionMergingFilter
TInputImage InputSpectralImageType
MeanShiftFilterType::LabelType InputLabelPixelType
RegionPruningFilterType::Pointer RegionPruningFilterPointerType
MeanShiftFilterPointerType m_MeanShiftFilter
MeanShiftFilterType::OutputLabelImageType InputLabelImageType
LabelImageRegionMergingFilter< InputLabelImageType, MeanShiftFilteredImageType, OutputLabelImageType, OutputClusteredImageType > RegionMergingFilterType
itk::SmartPointer< Self > Pointer
#define otbGetObjectMemberConstReferenceMacro(object, name, type)
MeanShiftSmoothingImageFilter< InputSpectralImageType, MeanShiftFilteredImageType, KernelType > MeanShiftFilterType
TOutputLabelImage OutputLabelImageType
Functor::ConnectedLabelFunctor< InputLabelPixelType > LabelFunctorType
std::string GetNameOfClass()
bool operator()(TInput &p1, TInput &p2)
ConnectedLabelFunctor Self
itk::ImageToImageFilter< TInputImage, TOutputLabelImage > Superclass
#define otbGetObjectMemberMacro(object, name, type)
#define otbSetObjectMemberMacro(object, name, type)
MeanShiftFilterType::Pointer MeanShiftFilterPointerType
itk::SmartPointer< Self > Pointer
TOutputClusteredImage OutputClusteredImageType
RelabelComponentFilterType::Pointer RelabelComponentFilterPointerType