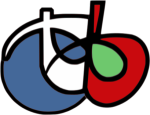 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbSpectralResponse_h
22 #define otbSpectralResponse_h
24 #include "itkDataObject.h"
25 #include <itkObjectFactory.h>
31 #include "itkImageRegionIterator.h"
54 template <
class TPrecision =
double,
class TValuePrecision =
double>
68 typedef std::pair<TPrecision, TValuePrecision>
PairType;
88 itkSetMacro(SensitivityThreshold, TPrecision);
89 itkGetConstMacro(SensitivityThreshold, TPrecision);
91 itkSetMacro(UsePosGuess,
bool);
92 itkGetConstMacro(UsePosGuess,
bool);
99 virtual unsigned int Size()
const;
105 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
132 return a.first < b.first;
193 #ifndef OTB_MANUAL_INSTANTIATION
itk::SmartPointer< Self > Pointer
std::pair< TPrecision, TValuePrecision > PairType
void SetFromImage(ImagePointerType image)
virtual unsigned int Size() const
void PrintSelf(std::ostream &os, itk::Indent indent) const override
itk::SmartPointer< FilterFunctionValuesType > FilterFunctionValuesPointerType
TValuePrecision ValuePrecisionType
itk::SmartPointer< Self > Pointer
FilterFunctionValuesPointerType GetFilterFunctionValues(double step=0.0025)
ImagePointerType GetImage(ImagePointerType image) const
ImageType::Pointer ImagePointerType
VectorPairType & GetResponse()
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
itk::SmartPointer< const Self > ConstPointer
This class contains the values of the filter function for the processed spectral band.
bool operator()(PairType a, PairType b)
void Load(const std::string &filename, ValuePrecisionType coefNormalization=1.0)
~SpectralResponse() override
otb::VectorImage< TValuePrecision, 2 > ImageType
TPrecision m_SensitivityThreshold
VectorPairType m_Response
ValuePrecisionType operator()(const PrecisionType &lambda)
itk::DataObject Superclass
std::vector< PairType > VectorPairType
otb::FilterFunctionValues FilterFunctionValuesType
itk::ImageRegionIterator< ImageType > IteratorType
IntervalType GetInterval()
This class represents the spectral response of an object (or a satellite band).
void operator=(const Self &)=delete
void SetPosGuessMin(const PrecisionType &lambda)
std::pair< TPrecision, TPrecision > IntervalType
void SetResponse(const VectorPairType &resp)
Creation of an "otb" vector image which contains metadata.