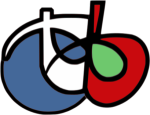 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
25 #include <unordered_set>
26 #include <unordered_map>
27 #include <boost/dynamic_bitset.hpp>
35 using Move = std::bitset<2>;
77 const std::size_t gridSizeX);
82 const std::size_t gridSizeX,
88 const std::size_t gridSizeX);
93 const std::size_t bboxWidth,
94 const std::size_t bboxHeight);
102 const std::size_t width,
103 const std::size_t height);
107 const std::size_t gridSizeX);
111 const std::size_t gridSizeX);
static void GenerateBorderCells(CellLists &borderCells, const Contour &contour, const CellIndex startCellId, const std::vcl_size_t gridSizeX)
static short GetMove10(const Move &m)
std::vcl_size_t CellIndex
std::unordered_set< CellIndex > CellLists
static CellIndex GridToBBox(const CellIndex gridId, const BoundingBox &bbox, const std::vcl_size_t gridSizeX)
static void Push0(Contour &contour)
static void CreateNewContour(Contour &newContour, const CellIndex startCellId, CellLists &setCells, const std::vcl_size_t bboxWidth, const std::vcl_size_t bboxHeight)
static void Push3(Contour &contour)
static Move GetMove2(ContourIndex idx, const Contour &contour)
static void MergeContour(Contour &mergedContour, BoundingBox &mergedBoundingBox, Contour &contour1, Contour &contour2, BoundingBox &bbox1, BoundingBox &bbox2, const CellIndex cid1, const CellIndex cid2, const std::vcl_size_t gridSizeX)
static void Push1(Contour &contour)
boost::dynamic_bitset<> Contour
static CellIndex BBoxToGrid(const CellIndex bboxId, const BoundingBox &bbox, const std::vcl_size_t gridSizeX)
static void Push2(Contour &contour)
static BoundingBox MergeBoundingBoxes(const BoundingBox &bb1, const BoundingBox &bb2)
boost::dynamic_bitset<>::size_type ContourIndex
static void GenerateBorderCellsForContourFusion(CellLists &borderCells, const Contour &contour, const CellIndex startCellId, const std::vcl_size_t gridSizeX, const BoundingBox &mergedBBox)
static void EIGHTNeighborhood(long int *neighborhood, const CellIndex id, const std::vcl_size_t width, const std::vcl_size_t height)