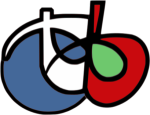 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
22 #include <vnl/vnl_vector.h>
23 #include <boost/algorithm/string.hpp>
34 std::vector<std::string>
string_split(
const std::string& s,
const std::string& sep);
46 unsigned int doy(
const std::tm& d);
62 auto tmp_doy =
doy(d);
97 std::vector<VectorType>
get_csv_profiles(
const std::string& fname,
int pos,
int ndates);
109 unsigned int nbDates);
111 int months(
const std::string& m);
vnl_vector< PrecisionType > VectorType
std::vector< VectorType > get_csv_profiles(const std::string &fname, int pos, int ndates)
DateVector parse_date_file(const std::string &df)
std::tm make_date(const std::string &d)
Makes a date (std::tm struct) from a string with format YYYYMMDD.
doy_multi_year(unsigned int d_prev_init)
std::string pad_int(int x)
return a string with a 0 at the front if the int is < 10
int delta_days(std::tm t1, std::tm t2)
Returns the difference in days between 2 time structures.
std::vector< std::string > string_split(const std::string &s, const std::string &sep)
Split a string using a given separator.
std::vector< std::tm > DateVector
int months(const std::string &m)
Map to convert months from text to int.
DateVector parse_dates_sirhyus(const std::string &fname)
std::vector< std::pair< long int, VectorType > > get_sirhyus_profiles(const std::string &df, unsigned int nbDates)
unsigned int doy(const std::tm &d)
Return the day of year.
unsigned int operator()(const std::tm &d)
bool operator<(std::tm x, std::tm y)
Compare 2 dates.
std::pair< DateVector, int > parse_dates_csv_file(const std::string &df, int year)