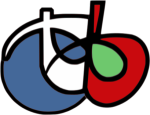 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
22 #ifndef otbGaborFilterGenerator_h
23 #define otbGaborFilterGenerator_h
25 #include "itkObject.h"
26 #include "itkObjectFactory.h"
72 template <
class TPrecision>
105 itkGetConstReferenceMacro(Radius,
RadiusType);
121 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
153 #ifndef OTB_MANUAL_INSTANTIATION
~GaborFilterGenerator() override
itk::Size< 2 > RadiusType
itk::SmartPointer< const Self > ConstPointer
itk::Array< PrecisionType > ArrayType
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
itk::SmartPointer< Self > Pointer
void PrintSelf(std::ostream &os, itk::Indent indent) const override
bool m_NeedToRegenerateFilter
void operator=(const Self &)=delete
GaborFilterGenerator Self
const ArrayType & GetFilter()
void Modified() const override