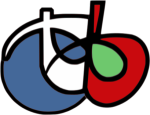 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbGenericRSTransform_hxx
22 #define otbGenericRSTransform_hxx
31 #include "ogr_spatialref.h"
37 template <
class TScalarType,
unsigned int NInputDimensions,
unsigned int NOutputDimensions>
56 template <
class TScalarType,
unsigned int NInputDimensions,
unsigned int NOutputDimensions>
60 itkDebugMacro(
"returning MapProjection address " << this->m_Transform);
61 if ((!m_TransformUpToDate) || (m_Transform.IsNull()))
63 itkExceptionMacro(<<
"m_Transform not up-to-date, call InstantiateTransform() first");
66 return this->m_Transform;
72 template <
class TScalarType,
unsigned int NInputDimensions,
unsigned int NOutputDimensions>
75 m_Transform = TransformType::New();
77 if (m_InputProjectionRef.empty() && m_InputImd !=
nullptr && m_InputImd->HasProjectedGeometry())
79 m_InputProjectionRef = m_InputImd->GetProjectionWKT();
85 otbMsgDevMacro(<<
" * Input metadata: " << ((m_InputImd ==
nullptr) ?
"Empty" :
"Full"));
86 otbMsgDevMacro(<<
" * Input projection: " << m_InputProjectionRef);
87 otbMsgDevMacro(<<
" * Output metadata: " << ((m_OutputImd ==
nullptr) ?
"Empty" :
"Full"));
88 otbMsgDevMacro(<<
" * Output projection: " << m_OutputProjectionRef);
93 m_InputTransform =
nullptr;
94 m_OutputTransform =
nullptr;
96 bool inputTransformIsSensor =
false;
97 bool inputTransformIsMap =
false;
98 bool outputTransformIsSensor =
false;
99 bool outputTransformIsMap =
false;
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
#define otbMsgDevMacro(x)