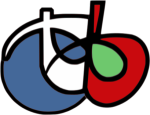 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbImageReference_h
22 #define otbImageReference_h
38 template <
typename TPrecision>
65 return "ImageReference";
154 return physicalPoint;
184 #ifndef OTB_MANUAL_INSTANTIATION
188 #endif // otbImageReference_h
PointType TransformPointToPhysicalPoint(const PointType &point) const
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
itk::Point< PrecisionType, 2 > OriginType
ImageReference & operator=(ImageReference const &rhs)
SpacingType m_Spacing
Spacing getter.
OriginType m_Origin
Spacing getter.
itk::Vector< PrecisionType, 2 > SpacingType
ImageReference(SpacingType const &spacing, OriginType const &origin, itk::Object const &holder)
itk::Object const & m_Holder
Spacing getter.
virtual void SetOrigin(OriginType _arg)
Origin getter.
const char * GetNameOfClass()
void SetOrigin(const TPrecision origin[Dimension])
Origin setter.
itk::Point< PrecisionType, 2 > PointType
void SetSpacing(const SpacingType &spacing)
Spacing getter.
void SetSpacing(const TPrecision spacing[Dimension])
Spacing getter.
ImageReference(itk::Object const &holder)
void TransformPointToPhysicalPoint(const PointType &point, PointType &physicalPoint) const