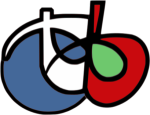 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbImageToProfileFilter_h
22 #define otbImageToProfileFilter_h
46 template <
class TInputImage,
class TOutputImage,
class TFilter,
class TParameter =
unsigned int>
77 itkSetMacro(ProfileSize,
unsigned int);
78 itkGetMacro(ProfileSize,
unsigned int);
85 itkSetMacro(OutputIndex,
unsigned int);
86 itkGetMacro(OutputIndex,
unsigned int);
98 itkGetObjectMacro(
Filter, FilterType);
101 void GenerateData(
void)
override;
104 void GenerateOutputInformation(
void)
override;
107 void GenerateInputRequestedRegion(
void)
override;
116 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
120 void operator=(
const Self&) =
delete;
138 #ifndef OTB_MANUAL_INSTANTIATION
unsigned int m_OutputIndex
Base class for all the filters producing an otbImageList.
Superclass::OutputImageListPointerType OutputImageListPointerType
FilterPointerType m_Filter
unsigned int m_ProfileSize
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
Base class to produce a profile of the response of a given filter for a range of parameter.
TInputImage InputImageType
FilterType::Pointer FilterPointerType
TOutputImage OutputImageType
ImageToImageListFilter< TInputImage, TOutputImage > Superclass
itk::SmartPointer< const Self > ConstPointer
Superclass::OutputImageListType OutputImageListType
ImageToProfileFilter Self
Superclass::InputImagePointer InputImagePointerType
ParameterType m_InitialValue
virtual void SetProfileParameter(ParameterType)
Base class for all the filters taking an image input to produce an image list.
itk::SmartPointer< Self > Pointer