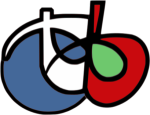 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbInverseLogPolarTransform_h
22 #define otbInverseLogPolarTransform_h
47 template <
class TScalarType>
83 itkGetConstReferenceMacro(Scale,
ScaleType);
103 this->m_FixedParameters = param;
112 return this->m_FixedParameters;
120 using Superclass::TransformVector;
121 OutputPointType TransformPoint(
const InputPointType& point)
const override;
128 OutputVectorType TransformVector(
const InputVectorType& vector)
const override;
135 OutputVnlVectorType TransformVector(
const InputVnlVectorType& vector)
const override;
145 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
149 void operator=(
const Self&) =
delete;
155 #ifndef OTB_MANUAL_INSTANTIATION
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.