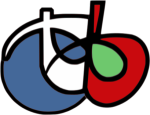 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
22 #ifndef otbKullbackLeiblerProfileImageFilter_h
23 #define otbKullbackLeiblerProfileImageFilter_h
28 #include "itkArray2D.h"
29 #include "itkVariableLengthVector.h"
42 template <
class TInput>
56 template <
class TInput2>
104 template <
class TInput1,
class TInput2,
class TOutput>
113 void SetRadius(
const unsigned char& min,
const unsigned char& max);
122 TOutput
operator()(
const TInput1& it1,
const TInput2& it2);
166 template <
class TInputImage1,
class TInputImage2,
class TOutputImage>
169 TInputImage1, TInputImage2, TOutputImage,
170 Functor::KullbackLeiblerProfile<typename itk::ConstNeighborhoodIterator<TInputImage1>, typename itk::ConstNeighborhoodIterator<TInputImage2>,
171 typename TOutputImage::PixelType>>
177 TInputImage1, TInputImage2, TOutputImage,
179 typename TOutputImage::PixelType>>
197 void operator=(
const Self&) =
delete;
202 #ifndef OTB_MANUAL_INSTANTIATION
TOutput operator()(const TInput1 &it1, const TInput2 &it2)
int InitSumAndMoments(const TInput &input, itk::Array2D< int > &mask)
std::vector< CumulantType > CumulantSet
virtual ~CumulantsForEdgeworthProfile()
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
void MakeMultiscaleProfile()
BinaryFunctorNeighborhoodVectorImageFilter< TInputImage1, TInputImage2, TOutputImage, Functor::KullbackLeiblerProfile< typename itk::ConstNeighborhoodIterator< TInputImage1 >, typename itk::ConstNeighborhoodIterator< TInputImage2 >, typename TOutputImage::PixelType > > Superclass
CumulantsForEdgeworthProfile()
Implements neighborhood-wise generic operation of two images being vector images.
std::vector< itk::Array2D< int > > m_mask
itk::SmartPointer< Self > Pointer
itk::VariableLengthVector< double > KL_profile(CumulantsForEdgeworthProfile< TInput2 > &cumulants)
KullbackLeiblerProfileImageFilter Self
unsigned char GetRadiusMin(void)
KullbackLeiblerProfileImageFilter()
itk::Vector< double, 4 > CumulantType
~KullbackLeiblerProfileImageFilter() override
unsigned char m_RadiusMax
unsigned char m_RadiusMin
Functor for KullbackLeiblerProfileImageFilter. Please refer to KullbackLeiblerProfileImageFilter.
int ReInitSumAndMoments(const TInput &input, itk::Array2D< int > &mask, int level)
CumulantSet::iterator Iterator
Helper class for KullbackLeiblerProfileImageFilter. Please refer to KullbackLeibleProfileImageFilter.
itk::SmartPointer< const Self > ConstPointer
int MakeSumAndMoments(const TInput &input, std::vector< itk::Array2D< int >> &mask)
int GetNumberOfComponentsPerPixel() const
unsigned char GetRadiusMax(void)
void SetRadius(const unsigned char &min, const unsigned char &max)
bool IsDataAvailable() const
virtual ~KullbackLeiblerProfile()
Implements neighborhood-wise the computation of KullbackLeibler profile over Edgeworth approximation.
CumulantType & GetCumulantAtScale(int i)