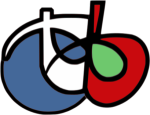 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
40 template <
typename Extents>
61 constexpr
bool is_unique() const noexcept {
return true ; }
62 constexpr
bool is_strided() const noexcept {
return true ; }
78 template <
typename Extents>
87 assert(r < this->
extents().rank());
89 for(
size_t k = 0 ; k < r; ++k)
90 res *= this->
extents().extent(k);
107 template <
typename Extents>
116 assert(r < this->
extents().rank());
118 for(
size_t k = r+1 ; k < this->
extents().rank(); ++k)
119 res *= this->
extents().extent(k);
138 template <
typename Extents>
157 assert(R < this->
extents().rank());
184 template <
template <
typename>
class LayoutPolicy>
188 template <
typename Extents>
193 using index_type =
typename LayoutPolicy<Extents>::index_type;
194 using LayoutPolicy<Extents>::LayoutPolicy;
197 template<
class... Indices>
200 std::array<
index_type, extents_type::rank()> idx{i...};
202 for (
size_t k = 0 ; k < extents_type::rank() ; ++k)
204 r += idx[k] * this->stride(k);
212 for (
size_t k = 0 ; k < Extents::rank() ; ++k)
213 r = std::max(r, this->stride(k) * this->
extents().
extent(k));
266 #endif // otbLayouts_h
constexpr bool is_contiguous() const noexcept
constexpr index_type operator()(Indices... i) const noexcept
constexpr index_type stride(const vcl_size_t r) const noexcept
typename LayoutPolicy< Extents >::index_type index_type
constexpr index_type stride(const vcl_size_t r) const noexcept
constexpr bool is_unique() const noexcept
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
typename Extents::index_type index_type
static constexpr bool is_always_strided() noexcept
constexpr index_type extent(vcl_size_t k) const noexcept
static constexpr bool is_always_contiguous() noexcept
constexpr bool is_strided() const noexcept
constexpr bool is_contiguous() const noexcept
constexpr stride_(Extents const &e, stride_t const &s) noexcept
typename root_layout_mapping< Extents >::index_type index_type
static constexpr bool is_always_contiguous() noexcept
constexpr Extents const & extents() const noexcept
constexpr root_layout_mapping() noexcept=default
~root_layout_mapping()=default
constexpr bool is_contiguous() const noexcept
std::array< index_type, Extents::rank()> stride_t
constexpr index_type stride(const vcl_size_t R) const noexcept
constexpr index_type required_span_size() const noexcept
static constexpr bool is_always_contiguous() noexcept
static constexpr bool is_always_unique() noexcept