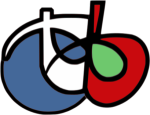 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbLogPolarTransform_h
22 #define otbLogPolarTransform_h
48 template <
class TScalarType>
84 itkGetConstReferenceMacro(Scale,
ScaleType);
104 this->m_FixedParameters = param;
113 return this->m_FixedParameters;
121 OutputPointType TransformPoint(
const InputPointType& point)
const override;
128 using Superclass::TransformVector;
129 OutputVectorType TransformVector(
const InputVectorType& vector)
const override;
136 OutputVnlVectorType TransformVector(
const InputVnlVectorType& vector)
const override;
146 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
150 void operator=(
const Self&) =
delete;
156 #ifndef OTB_MANUAL_INSTANTIATION
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.