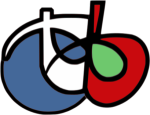 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbMeanShiftConnectedComponentSegmentationFilter_h
22 #define otbMeanShiftConnectedComponentSegmentationFilter_h
27 #include "itkConnectedComponentFunctorImageFilter.h"
29 #include "itkRelabelComponentImageFilter.h"
58 template <
class TVInputImage,
class TMaskImage,
class TLabelImage>
65 typedef itk::ImageToImageFilter<TVInputImage, TLabelImage>
Superclass;
110 itkSetStringMacro(MaskExpression);
113 itkGetStringMacro(MaskExpression);
116 itkSetStringMacro(ConnectedComponentExpression);
119 itkGetStringMacro(ConnectedComponentExpression);
148 #ifndef OTB_MANUAL_INSTANTIATION
VectorImageType::Pointer VectorImagePointerType
VectorImageType::PixelType VectorImagePixelType
static const unsigned int InputImageDimension
void GenerateInputRequestedRegion() override
TVInputImage VectorImageType
std::string m_MaskExpression
itk::ConnectedComponentFunctorImageFilter< VectorImageType, LabelImageType, FunctorType, MaskImageType > ConnectedComponentFilterType
Functor::ConnectedComponentMuParserFunctor< VectorImagePixelType > FunctorType
itkGetObjectMacro(MeanShiftFilter, MeanShiftFilterType)
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
itk::RelabelComponentImageFilter< LabelImageType, LabelImageType > RelabelComponentFilterType
MeanShiftFilterPointerType m_MeanShiftFilter
MeanShiftConnectedComponentSegmentationFilter Self
MeanShiftFilterType::Pointer MeanShiftFilterPointerType
MeanShiftConnectedComponentSegmentationFilter()
~MeanShiftConnectedComponentSegmentationFilter() override
itk::SmartPointer< Self > Pointer
void GenerateData() override
TLabelImage LabelImageType
unsigned int ObjectSizeType
itk::ImageToImageFilter< TVInputImage, TLabelImage > Superclass
itk::SmartPointer< const Self > ConstPointer
ObjectSizeType m_MinimumObjectSize
otb::MeanShiftSmoothingImageFilter< VectorImageType, VectorImageType > MeanShiftFilterType
[internal] Helper class to perform connected component segmentation on an input image,
otb::MaskMuParserFilter< VectorImageType, MaskImageType > MaskMuParserFilterType
std::string m_ConnectedComponentExpression
itk::SmartPointer< Self > Pointer