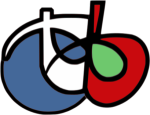 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbMetadataSupplierInterface_hxx
22 #define otbMetadataSupplierInterface_hxx
42 return boost::lexical_cast<T>(ret);
44 catch (boost::bad_lexical_cast&)
61 return boost::lexical_cast<T>(ret);
63 catch (boost::bad_lexical_cast&)
79 std::vector<T> output;
81 const range_type parts =
split_on(filt_value, sep);
82 for (
auto const& part : parts)
85 std::string strPart = to<std::string>(part,
"casting string_view to std::string");
92 output.push_back(boost::lexical_cast<T>(strPart));
94 catch (boost::bad_lexical_cast&)
99 if ((size >= 0) && (output.size() != (
size_t)size))
part_range< splitter_on_delim > split_on(String const &str, char delim)
OTBCommon_EXPORT string_view lstrip(string_view const &v, string_view const &c)
returns a string_view with the leading characters removed
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
#define otbGenericExceptionMacro(T, x)
OTBCommon_EXPORT string_view rstrip(string_view const &v, string_view const &c)
returns a string_view with the ending characters removed