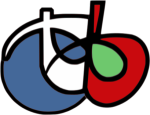 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
41 NotNull (std::nullptr_t) =
delete;
45 constexpr T
get()
const
51 constexpr
operator T()
const {
return get(); }
53 constexpr decltype(
auto) operator*()
const {
return *
get(); }
62 void operator[](std::ptrdiff_t)
const =
delete;
70 #endif // otbNotNull_h
NotNull & operator--()=delete
NotNull & operator-=(std::ptrdiff_t)=delete
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
NotNull & operator+=(std::ptrdiff_t)=delete
constexpr T operator->() const
NotNull & operator++()=delete
void operator[](std::ptrdiff_t) const =delete
NotNull & operator=(std::nullptr_t)=delete