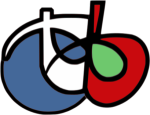 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
22 #ifndef QuadraticallyConstrainedSimpleSolver_H_
23 #define QuadraticallyConstrainedSimpleSolver_H_
25 #include "itkObjectFactory.h"
26 #include "itkLightObject.h"
27 #include "itkNumericTraits.h"
28 #include <vnl/vnl_matrix.h>
29 #include "vnl/algo/vnl_solve_qp.h"
65 template <
class ValueType>
94 Cost_Function_weighted_musig
104 return m_MeanInOverlaps;
115 return m_StandardDeviationInOverlaps;
126 return m_AreaInOverlaps;
137 return m_MeanOfProductsInOverlaps;
144 return m_OutputCorrectionModel;
155 m_WeightOfStandardDeviationTerm = weight;
159 return m_WeightOfStandardDeviationTerm;
169 oft = Cost_Function_mu;
173 oft = Cost_Function_musig;
177 oft = Cost_Function_rmse;
181 oft = Cost_Function_weighted_musig;
191 void CheckInputs(
void)
const;
194 void DFS(std::vector<bool>& marked,
unsigned int s)
const;
197 const DoubleMatrixType GetQuadraticObjectiveMatrix(
const DoubleMatrixType& areas,
const DoubleMatrixType& means,
const DoubleMatrixType& stds,
198 const DoubleMatrixType& mops);
201 const DoubleMatrixType ExtractMatrix(
const RealMatrixType& mat,
const ListIndexType& idx);
220 #ifndef OTB_MANUAL_INSTANTIATION
vnl_vector< ValueType > RealVectorType
itk::SmartPointer< const Self > ConstPointer
itk::LightObject Superclass
void SetMeanAndStandardDeviationBased()
vnl_matrix< double > DoubleMatrixType
const RealMatrixType GetMeanOfProductsInOverlaps()
RealMatrixType m_MeanOfProductsInOverlaps
void SetStandardDeviationInOverlaps(const RealMatrixType &matrix)
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
const RealMatrixType GetStandardDeviationInOverlaps()
const RealVectorType GetOutputCorrectionModel()
vnl_vector< double > DoubleVectorType
void SetMeanInOverlaps(const RealMatrixType &matrix)
Solves the optimisation problem for radiometric harmonisation of multiple overlapping images.
ObjectiveFunctionType oft
void SetWeightedMeanAndStandardDeviationBased()
const RealMatrixType GetAreaInOverlaps()
std::vector< unsigned int > ListIndexType
void SetMeanOfProductsInOverlaps(const RealMatrixType &matrix)
RealVectorType m_OutputCorrectionModel
ValueType GetWeightOfStandardDeviationTerm()
itk::SmartPointer< Self > Pointer
const RealMatrixType GetMeanInOverlaps()
void SetAreaInOverlaps(const RealMatrixType &matrix)
void SetWeightOfStandardDeviationTerm(ValueType weight)
RealMatrixType m_MeanInOverlaps
ValueType m_WeightOfStandardDeviationTerm
RealMatrixType m_StandardDeviationInOverlaps
RealMatrixType m_AreaInOverlaps
void Solve(const GCPsContainerType &gcpContainer, double &rmsError, Projection::RPCParam &outputParams)
vnl_matrix< ValueType > RealMatrixType
QuadraticallyConstrainedSimpleSolver Self