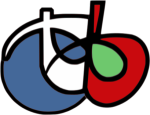 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbSavitzkyGolayInterpolationFunctor_h
22 #define otbSavitzkyGolayInterpolationFunctor_h
56 template <
unsigned int Radius,
class TSeries,
class TDates,
class TWeight = TSeries,
unsigned int Degree = 2>
65 static const unsigned int nbDates = TSeries::Dimension;
92 for (
unsigned int i = 0; i <
m_DoySeries.Size(); ++i)
100 unsigned int firstSample = Radius;
101 unsigned int lastSample =
nbDates - Radius - 1;
103 for (
unsigned int i = 0; i < firstSample; ++i)
104 outSeries[i] = series[i];
105 for (
unsigned int i = lastSample + 1; i <
nbDates; ++i)
106 outSeries[i] = series[i];
108 for (
unsigned int i = firstSample; i <= lastSample; ++i)
114 for (
unsigned int j = 0; j <= 2 * Radius; ++j)
116 tmpInSeries[j] = series[i + j - Radius];
125 outSeries[i] = tmpOutSeries[Radius];
otb::PolynomialTimeSeries< Degree, CoefficientPrecisionType > TSFunctionType
void SetDates(const TDates doy)
itk::FixedArray< WeightType, InterpolatedLength > InterpolatedWeightType
TWeight::ValueType WeightType
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
virtual ~SavitzkyGolayInterpolationFunctor()
Destructor.
double CoefficientPrecisionType
static const unsigned int InterpolatedLength
void SetWeights(const TWeight weights)
static const unsigned int nbDates
otb::Functor::TimeSeriesLeastSquareFittingFunctor< InterpolatedSeriesType, TSFunctionType, InterpolatedDatesType, InterpolatedWeightType > TLSFunctorType
itk::FixedArray< ValueType, InterpolatedLength > InterpolatedSeriesType
TSeries operator()(const TSeries &series) const
unsigned int doy(const std::tm &d)
Return the day of year.
TSeries::ValueType ValueType
Implements a least squares fitting of a time profile.
itk::FixedArray< DateType, InterpolatedLength > InterpolatedDatesType
SavitzkyGolayInterpolationFunctor()
Constructor.
TDates::ValueType DateType
void SetDates(const TDateType &doy)
void SetWeights(const TWeightType &weights)