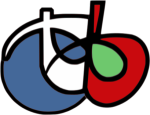 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
20 #ifndef otbGDALRPCTransformer_h
21 #define otbGDALRPCTransformer_h
67 GDALRPCTransformer(
double LineOffset,
double SampleOffset,
double LatOffset,
double LonOffset,
double HeightOffset,
68 double LineScale,
double SampleScale,
double LatScale,
double LonScale,
double HeightScale,
69 const double (&LineNum)[20],
const double (&LineDen)[20],
const double (&SampleNum)[20],
const double (&SampleDen)[20],
bool useDEM);
135 void SetOption(
const std::string& Name,
const std::string& Value);
Observer design pattern to keep track of DEM configuration changes.
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
Coefficients for RPC model (quite similar to GDALRPCInfo)