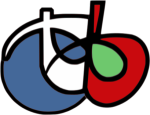 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbReduceSpectralResponse_h
22 #define otbReduceSpectralResponse_h
25 #include "itkDataObject.h"
26 #include <itkObjectFactory.h>
63 template <
class TSpectralResponse,
class TRSR>
78 typedef typename InputSpectralResponseType::PairType
PairType;
112 itkSetMacro(ReflectanceMode,
bool);
113 itkGetConstMacro(ReflectanceMode,
bool);
116 virtual bool Clear();
119 void PrintSelf(std::ostream& os, itk::Indent indent)
const override;
131 void LoadInputsFromFiles(
const std::string& spectralResponseFile,
const std::string& RSRFile,
const unsigned int nbRSRBands,
167 #ifndef OTB_MANUAL_INSTANTIATION
This class computes the reduced spectral response of each band of a sensor.
InputSpectralResponseType::VectorPairType VectorPairType
InputRSRType::PrecisionType PrecisionType
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
InputRSRType::RSRVectorType RSRVectorType
ReduceSpectralResponse Self
InputSpectralResponsePointerType m_ReduceResponse
InputSpectralResponseType::Pointer InputSpectralResponsePointerType
void PrintSelf(std::ostream &os, itk::Indent indent) const override
itk::DataObject Superclass
~ReduceSpectralResponse() override
void operator=(const Self &)=delete
void LoadInputsFromFiles(const std::string &spectralResponseFile, const std::string &RSRFile, const unsigned int nbRSRBands, ValuePrecisionType coefNormSpectre=1.0, ValuePrecisionType coefNormRSR=1.0)
InputRSRType::ValuePrecisionType ValuePrecisionType
itkGetObjectMacro(ReduceResponse, InputSpectralResponseType)
ValuePrecisionType operator()(const unsigned int numBand)
InputSpectralResponseType::PairType PairType
std::vector< ValuePrecisionType > ReduceSpectralResponseVectorType
InputSpectralResponsePointerType m_InputSpectralResponse
InputRSRType::Pointer InputRSRPointerType
itk::SmartPointer< const Self > ConstPointer
itk::SmartPointer< Self > Pointer
InputRSRPointerType m_InputSatRSR
TSpectralResponse InputSpectralResponseType