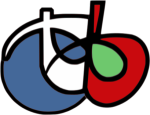 |
OTB
9.0.0
Orfeo Toolbox
|
Go to the documentation of this file.
21 #ifndef otbSarSensorModel_h
22 #define otbSarSensorModel_h
53 std::string productType,
56 unsigned int polynomial_degree = 8);
195 double heightAboveEllipsoid,
238 std::tuple<TimeType, OrbitIterator, OrbitIterator>
257 bool Deburst(std::vector<std::pair<unsigned long, unsigned long>>& lines,
258 std::pair<unsigned long, unsigned long>& samples,
259 bool onlyValidSample =
false);
273 bool BurstExtraction(
const unsigned int burst_index, std::pair<unsigned long,unsigned long> & lines,
274 std::pair<unsigned long,unsigned long> & samples,
bool allPixels =
false);
292 std::vector<std::pair<unsigned long,unsigned long> >& samplesBursts,
293 unsigned int & linesOffset,
unsigned int first_burstInd,
294 bool inputWithInvalidPixels=
false);
310 bool Overlap(std::pair<unsigned long, unsigned long>& linesUp, std::pair<unsigned long, unsigned long>& linesLow,
311 std::pair<unsigned long, unsigned long>& samplesUp, std::pair<unsigned long, unsigned long>& samplesLow,
unsigned int burstIndUp,
312 bool inputWithInvalidPixels =
false);
328 unsigned long deburstLine,
329 unsigned long & imageLine);
340 unsigned long imageLine,
341 unsigned long & deburstLine);
395 const TimeType & azimuthTime)
const;
400 const std::vector<CoordinateConversionRecord> & records)
const;
406 std::function<
double(
double,
double)> heightFunction)
const;
411 itk::Point<double, 3>
EcefToWorld(
const itk::Point<double, 3> & ecefPoint)
const;
414 itk::Point<double, 3>
WorldToEcef(
const itk::Point<double, 3> & worldPoint)
const;
std::tuple< TimeType, OrbitIterator, OrbitIterator > ZeroDopplerTimeLookupInternal(Point3DType const &ecefGround) const
LineSampleYZ Doppler0ToLineSampleYZ(Point3DType const &ecefGround, ZeroDopplerInfo const &zdi, double rangeTime) const
double AzimuthTimeToLine(const TimeType &azimuthTime) const
void WorldToLineSampleYZ(const Point3DType &inGeoPoint, Point2DType &cr, Point2DType &yz) const
OrbitIterator searchLagrangianNeighbourhood(TimeType azimuthTime) const
SarSensorModel(std::string productType, SARParam sarParam, Projection::GCPParam gcps, unsigned int polynomial_degree=8)
void WorldToLineSample(const Point3DType &inGeoPoint, Point2DType &outLineSample) const
itk::Point< double, 3 > WorldToEcef(const itk::Point< double, 3 > &worldPoint) const
const GCP & findClosestGCP(const Point2DType &imPt, const Projection::GCPParam &gcpParam) const
void OptimizeTimeOffsetsFromGcps()
The "otb" namespace contains all Orfeo Toolbox (OTB) classes.
unsigned int m_polynomial_degree
static bool ImageLineToDeburstLine(const std::vector< std::pair< unsigned long, unsigned long > > &lines, unsigned long imageLine, unsigned long &deburstLine)
bool LineToSatPositionAndVelocity(double line, Point3DType &satellitePosition, Point3DType &satelliteVelocity) const
std::string m_ProductType
ZeroDopplerInfo ZeroDopplerLookup(Point3DType const &inEcefPoint) const
bool WorldToSatPositionAndVelocity(const Point3DType &inGeoPoint, Point3DType &satellitePosition, Point3DType &satelliteVelocity) const
std::vector< Orbit >::const_iterator OrbitIterator
std::pair< Point3DType, Vector3DType > interpolateSensorPosVel(TimeType azimuthTime) const
otb::GeocentricTransform< otb::TransformDirection::INVERSE, double >::Pointer m_EcefToWorldTransform
bool Overlap(std::pair< unsigned long, unsigned long > &linesUp, std::pair< unsigned long, unsigned long > &linesLow, std::pair< unsigned long, unsigned long > &samplesUp, std::pair< unsigned long, unsigned long > &samplesLow, unsigned int burstIndUp, bool inputWithInvalidPixels=false)
double SlantRangeToGroundRange(double slantRange, const TimeType &azimuthTime) const
SarSensorModel & operator=(const SarSensorModel &)=delete
static void DeburstLineToImageLine(const std::vector< std::pair< unsigned long, unsigned long > > &lines, unsigned long deburstLine, unsigned long &imageLine)
double CalculateRangeTime(Point3DType const &ecefGround, Point3DType const &sensorPos) const
Projection::GCPParam m_GCP
bool WorldToAzimuthRangeTime(const Point3DType &inGeoPoint, TimeType &azimuthTime, double &rangeTime, Point3DType &sensorPos, Vector3DType &sensorVel) const
itk::Point< double, 3 > EcefToWorld(const itk::Point< double, 3 > &ecefPoint) const
otb::GeocentricTransform< otb::TransformDirection::FORWARD, double >::Pointer m_WorldToEcefTransform
bool BurstExtraction(const unsigned int burst_index, std::pair< unsigned long, unsigned long > &lines, std::pair< unsigned long, unsigned long > &samples, bool allPixels=false)
bool Deburst(std::vector< std::pair< unsigned long, unsigned long >> &lines, std::pair< unsigned long, unsigned long > &samples, bool onlyValidSample=false)
void LineSampleHeightToWorld(const Point2DType &imPt, double heightAboveEllipsoid, Point3DType &worldPt) const
itk::Point< double, 3 > Vector3DType
void UpdateImageMetadata(ImageMetadata &imd)
bool DeburstAndConcatenate(std::vector< std::pair< unsigned long, unsigned long > > &linesBursts, std::vector< std::pair< unsigned long, unsigned long > > &samplesBursts, unsigned int &linesOffset, unsigned int first_burstInd, bool inputWithInvalidPixels=false)
LagrangianOrbitInterpolator m_OrbitInterpolator
Point3DType projToSurface(const GCP &gcp, const Point2DType &imPt, std::function< double(double, double)> heightFunction) const
TimeType ZeroDopplerTimeLookup(Point3DType const &ecefGround) const
double ApplyCoordinateConversion(double in, const TimeType &azimuthTime, const std::vector< CoordinateConversionRecord > &records) const
This structure handles the list of the GCP parameters.
void LineSampleToWorld(const Point2DType &imPt, Point3DType &worldPt) const
TimeType LineToAzimuthTime(double line) const
virtual ~SarSensorModel()=default
itk::Point< double, 2 > Point2DType
DurationType m_AzimuthTimeOffset
itk::Point< double, 3 > Point3DType
This GCP class is used to manage the GCP parameters in OTB.